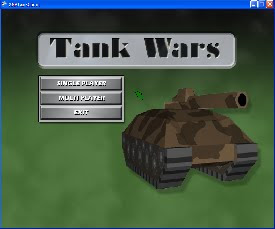
In a previous post I put together a simple multiplayer tank game with GDI+. But, I decided to change to XNA when I hit a limitation with the game sound. What a perfect opportunity to make it 3D!
There are many advantages to using XNA 4.0, especially if you want to play your game on the xbox 360 or Zune. But, keep in mind that if you want to play it on the XBox 360 you will need to purchase a Developers Membership at App Hub
Content Creation
The first task is to create the tank model and turret using Artismo 3D. If you want a copy just let me know by commenting on this page. Or send me your e-mail by using my contact page here.
The tank in Artismo:
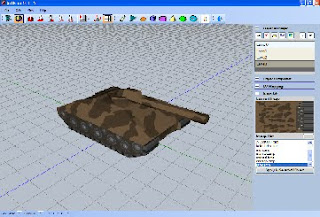
In Artismo I can create the models and the artwork in one application. It even does animation for characters. And, supports plug-in architecture to incorporate project-specific processes. For example, a level editor plug-in could be incorporated into the tool to produce the levels for your game.
Tank Movement
Moving around a 3D object is a lot easier than in GDI+. In GDI+ you're only drawing a square bitmap and everything is centered on the upper left corner so you have to move the image up there, rotate it, and put it back. In 3D you have a matrix and XNA provides really simple functions to rotate or translate the object around. If you ever used Managed DirectX for Windows it is very similar code.
User Interface
In the first version I had regular windows textbox controls and buttons. Not in XNA! You'll have to code them or get a GUI library on the internet. They're not that hard to code though. But, the textbox control does take a little work. If you do a few searches on the internet you can find some examples of doing it. The font support was really easy as well.
Client/Server Protocol
On the server side, there were a few changes because I had to send more data now. Instead of just a position point in 2D and a rotation amount, I changed it to send the tank matrix or transform. It is possible to do it with the original data but it was much easier to pass back and forth the exact data I needed instead of trying to derive the matrix from pieces of information.
Sound
Sound was a lot easier! I can play multiple sounds at the same time and XNA even supports stereo functionality so if a tank blasts off a round to your right you can hear it in the right speaker. I didn't take it that far but atleast it is good to know that it supports it.
Collision
I used a small 3D ball for the bullet. To do the collision I created a Bounding Box roughly the same size of the tank. Then, I move the position of the bullet into the model's space and create a Bounding Sphere for it and do collision against the two. All of the collision code is built into XNA. It looks like this:
BoundingSphere sphere = new BoundingSphere(bulletpoint, 0.2f);
// create a bounding box for each object.
BoundingBox bbox = new BoundingBox(new Vector3(-3, 0, -3), new Vector3(3, 3, 3));
bbox.Intersects(ref sphere, out result);
if (result)
{
od.Health -= 1;
return true;
}
Conclusion
The end result is a 3D version of my original tank game that can be played on multiple computers. Some extra features that could be tossed in there is chatting, explosions, etc. That's as far as I'm going with this game.
Tank Wars Single Player with 20 NPC Tanks:
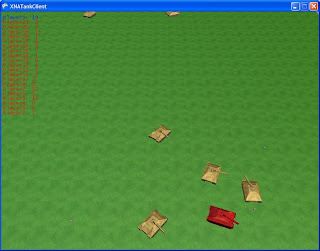
Next time we'll discuss level design and make some plug-ins for Artismo to produce the levels.
Happy Coding!
Jason
Hey that looks pretty awesome, btw your link's dead, can you post the code somewhere for us to see ? thanks
ReplyDelete